Monday 26 August 2013
Saturday 17 August 2013
Java Features
pankaj saxena
01:59
0
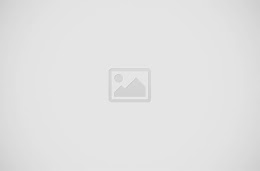
- Here we list the basic features that make Java a powerful and popular programming language:
- Platform Independence
- The Write-Once-Run-Anywhere ideal has not been achieved (tuning for different platforms usually required), but closer than with other languages.
- Object Oriented
- Object oriented throughout - no coding outside of class definitions, including main().
- An extensive class library available in the core language
packages.
- Compiler/Interpreter Combo
- Code is compiled to bytecodes that are interpreted by a Java virtual machines (JVM) .
- This provides portability to any machine for which a virtual machine has been written.
- The two steps of compilation and interpretation allow for
extensive code checking and improved security.
- Robust
- Exception handling built-in, strong type checking (that
is, all data must be declared an explicit type), local variables
must be initialized.
- Exception handling built-in, strong type checking (that
is, all data must be declared an explicit type), local variables
must be initialized.
- Several dangerous features of C &
C++ eliminated:
- No memory pointers
- No preprocessor
- Array index limit checking
- Automatic Memory Management
- Automatic garbage collection - memory management handled
by JVM.
- Automatic garbage collection - memory management handled
by JVM.
- Security
- No memory pointers
- Programs runs inside the virtual machine sandbox.
- Array index limit checking
- Code pathologies reduced by
- bytecode verifier - checks classes after loading
- class loader - confines objects to unique namespaces. Prevents loading a hacked "java.lang.SecurityManager" class, for example.
- security manager - determines what resources
a class can access such as reading and writing to the
local disk.
- Dynamic Binding
- The linking of data and methods to where they are located, is done at run-time.
- New classes can be loaded while a program is running. Linking is done on the fly.
- Even if libraries are recompiled, there is no need to recompile
code that uses classes in those libraries.
This differs from C++, which uses static binding. This can result in fragile classes for cases where linked code is changed and memory pointers then point to the wrong addresses.
- Good Performance
- Interpretation of bytecodes slowed performance in early
versions, but advanced virtual machines with adaptive and
just-in-time compilation and other techniques now typically
provide performance up to 50% to 100% the speed of C++ programs.
- Interpretation of bytecodes slowed performance in early
versions, but advanced virtual machines with adaptive and
just-in-time compilation and other techniques now typically
provide performance up to 50% to 100% the speed of C++ programs.
- Threading
- Lightweight processes, called threads, can easily be spun off to perform multiprocessing.
- Can take advantage of multiprocessors where available
- Great for multimedia displays.
- Built-in Networking
- Java was designed with networking in mind and comes with many classes to develop sophisticated Internet communications.
Tags
Continue Reading
Monday 12 August 2013
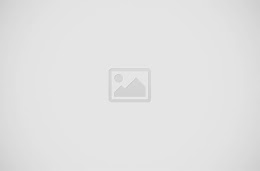
Difference between JAVA and C++
C++ | Java |
---|---|
Strongly influenced by C syntax, with Object-Oriented features added. | Strongly influenced by C++/C syntax. |
Compatible with C source code, except for a few corner cases. | Provides the Java Native Interface and recently Java Native Access as a way to directly call C/C++ code. |
Write once, compile anywhere (WOCA). | Write once, run anywhere / everywhere (WORA / WORE). |
Allows procedural programming, functional programming, object-oriented programming, generic programming, and template metaprogramming. Favors a mix of paradigms. | Strongly encourages exclusive use of the object-oriented programming paradigm. Includes support for generic programming and creation of scripting languages. |
Runs as native executable machine code for the target instruction set(s). | Runs in a virtual machine. |
Provides object types and type names. Allows reflection through RTTI. | Is reflective, allowing metaprogramming and dynamic code generation at runtime. |
Has multiple binary compatibility standards (commonly Microsoft (for MSVC compiler) and Itanium/GNU (for virtually all other compilers)). | Has a single, OS- and compiler-independent binary compatibility standard, allowing runtime check of correctness of libraries. |
Optional automated bounds checking (e.g., the at() method in vector and string containers). | All operations are required to be Bound-checked by all compliant distributions of Java. HotSpot can remove bounds checking. |
Supports native unsigned arithmetic. | No native support for unsigned arithmetic. |
Standardized minimum limits for all numerical types, but the actual
sizes are implementation-defined. Standardized types are available
through the standard library <cstdint> . |
Standardized limits and sizes of all primitive types on all platforms. |
Pointers, references, and pass-by-value are supported for all types (primitive or user-defined). | All types (primitive types and reference types) are always passed by value.[1] |
Memory management can be done explicitly through new / delete ,
by automatic scope-based resource management (RAII), or by smart
pointers. Supports deterministic destruction of objects. Garbage
collection ABI standardized in C++11, though compilers are not required
to implement garbage collection. |
Automatic garbage collection. Supports a non-deterministic finalize() method whose use is not recommended.[2] |
Resource management can be done manually or by automatic scope-based resource management (RAII). | Resource management must be done manually, or automatically via finalisers, though this is generally discouraged. Has try-with-resources for automatic scope-based resource management (version 7 onwards). |
Supports classes, structs (POD-types), and unions, and can allocate them on the heap or the stack. | Classes are allocated on the heap. Java SE 6 optimizes with escape analysis to allocate some objects on the stack. |
Allows explicitly overriding types as well as some implicit narrowing conversions (for compatibility with C). | Rigid type safety except for widening conversions. |
The C++ Standard Library
was designed to have a limited scope and functionality but includes
language support, diagnostics, general utilities, strings, locales,
containers, algorithms, iterators,
numerics, input/output, random number generators, regular expression
parsing, threading facilities, type traits (for static type
introspection) and Standard C Library. The Boost library offers more functionality including network I/O.
A rich amount of third-party libraries exist for GUI and other functionalities like: ACE, Crypto++, various XMPP Instant Messaging (IM) libraries,[3] OpenLDAP, Qt, gtkmm. |
The standard library has grown with each release. By version 1.6, the library included support for locales, logging, containers and iterators, algorithms, GUI programming (but not using the system GUI), graphics, multi-threading, networking, platform security, introspection, dynamic class loading, blocking and non-blocking I/O. It provided interfaces or support classes for XML, XSLT, MIDI, database connectivity, naming services (e.g. LDAP), cryptography, security services (e.g. Kerberos), print services, and web services. SWT offers an abstraction for platform-specific GUIs. |
Operator overloading for most operators. Preserving meaning (semantics) is highly recommended. | Operators are not overridable. The language overrides + and += for the String class. |
Single and Multiple inheritance of classes, including virtual inheritance. | Single inheritance of classes. Supports multiple inheritance via the Interfaces construct, which is equivalent to a C++ class composed of virtual methods. |
Compile-time templates. Allows for Turing complete meta-programming. | Generics are used to achieve basic type-parametrization, but they do not translate from source code to byte code due to the use of type erasure by the compiler. |
Function pointers, function objects, lambdas (in C++11), and interfaces. | References to functions achieved via the reflection API. OOP idioms using Interfaces, such as Adapter, Observer, and Listener are generally preferred over direct references to methods. |
No standard inline documentation mechanism. Third-party software (e.g. Doxygen) exists. | Extensive Javadoc documentation standard on all system classes and methods. |
const keyword for defining immutable variables and
member functions that do not change the object. Const-ness is propagated
as a means to enforce, at compile-time, correctness of the code with
respect to mutability of objects (see const-correctness). |
final provides a version of const , equivalent to type* const pointers for objects and const for primitive types. Immutability of object members achieved through read-only interfaces and object encapsulation. |
Supports the goto statement. |
Supports labels with loops and statement blocks. |
Source code can be written to be platform-independent (can be compiled for Windows, BSD, Linux, Mac OS X, Solaris, etc., without modification) and written to take advantage of platform-specific features. Typically compiled into native machine code, must be re-compiled for each target platform. | Compiled into byte code for the JVM. Byte code is dependent on the Java platform, but is typically independent of operating system specific features. |
Tags
Continue Reading
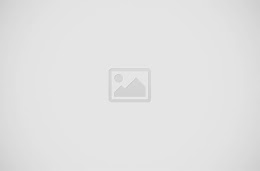
Difference Between Procedure Oriented Programming (POP) & Object Oriented Programming (OOP)
Procedure Oriented Programming |
Object Oriented Programming | |
---|---|---|
Divided Into |
In POP, program is divided into small parts called functions. | In OOP, program is divided into parts called objects. |
Importance |
In POP,Importance is not given to data but to functions as well as sequence of actions to be done. | In OOP, Importance is given to the data rather than procedures or functions because it works as a real world. |
Approach |
POP follows Top Down approach. | OOP follows Bottom Up approach. |
Access Specifiers |
POP does not have any access specifier. | OOP has access specifiers named Public, Private, Protected, etc. |
Data Moving | In POP, Data can move freely from function to function in the system. | In OOP, objects can move and communicate with each other through member functions. |
Expansion | To add new data and function in POP is not so easy. | OOP provides an easy way to add new data and function. |
Data Access | In POP, Most function uses Global data for sharing that can be accessed freely from function to function in the system. | In OOP, data can not move easily from function to function,it can be kept public or private so we can control the access of data. |
Data Hiding | POP does not have any proper way for hiding data so it is less secure. | OOP provides Data Hiding so provides more security. |
Overloading |
In POP, Overloading is not possible. | In OOP, overloading is possible in the form of Function Overloading and Operator Overloading. |
Examples | Example of POP are : C, VB, FORTRAN, Pascal. | Example of OOP are : C++, JAVA, VB.NET, C#.NET. |
Tags
Continue Reading
pankaj saxena
02:31
0
JAVA History
James Gosling, Mike Sheridan, and Patrick Naughton initiated the Java language project in June 1991.[12] Java was originally designed for interactive television, but it was too advanced for the digital cable television industry at the time.[13] The language was initially called Oak after an oak tree that stood outside Gosling's office; it went by the name Green later, and was later renamed Java, from Java coffee, said to be consumed in large quantities by the language's creators.[citation needed] Gosling aimed to implement a virtual machine and a language that had a familiar C/C++ style of notation.[14]Sun Microsystems released the first public implementation as Java 1.0 in 1995.[1] It promised "Write Once, Run Anywhere" (WORA), providing no-cost run-times on popular platforms. Fairly secure and featuring configurable security, it allowed network- and file-access restrictions. Major web browsers soon incorporated the ability to run Java applets within web pages, and Java quickly became popular. With the advent of Java 2 (released initially as J2SE 1.2 in December 1998 – 1999), new versions had multiple configurations built for different types of platforms. For example, J2EE targeted enterprise applications and the greatly stripped-down version J2ME for mobile applications (Mobile Java). J2SE designated the Standard Edition. In 2006, for marketing purposes, Sun renamed new J2 versions as Java EE, Java ME, and Java SE, respectively.
In 1997, Sun Microsystems approached the ISO/IEC JTC1 standards body and later the Ecma International to formalize Java, but it soon withdrew from the process.[15] Java remains a de facto standard, controlled through the Java Community Process.[16] At one time, Sun made most of its Java implementations available without charge, despite their proprietary software status. Sun generated revenue from Java through the selling of licenses for specialized products such as the Java Enterprise System. Sun distinguishes between its Software Development Kit (SDK) and Runtime Environment (JRE) (a subset of the SDK); the primary distinction involves the JRE's lack of the compiler, utility programs, and header files.
On November 13, 2006, Sun released much of Java as free and open source software, (FOSS), under the terms of the GNU General Public License (GPL). On May 8, 2007, Sun finished the process, making all of Java's core code available under free software/open-source distribution terms, aside from a small portion of code to which Sun did not hold the copyright.[17]
Sun's vice-president Rich Green said that Sun's ideal role with regards to Java was as an "evangelist."[18] Following Oracle Corporation's acquisition of Sun Microsystems in 2009–2010, Oracle has described itself as the "steward of Java technology with a relentless commitment to fostering a community of participation and transparency".[19] This did not hold Oracle, however, from filing a lawsuit against Google shortly after that for using Java inside the Android SDK (see Google section below). Java software runs on laptops to data centers, game consoles to scientific supercomputers. There are 930 million Java Runtime Environment downloads each year and 3 billion mobile phones run Java.[20] On April 2, 2010, James Gosling resigned from Oracle.[21]
Tags
Continue Reading